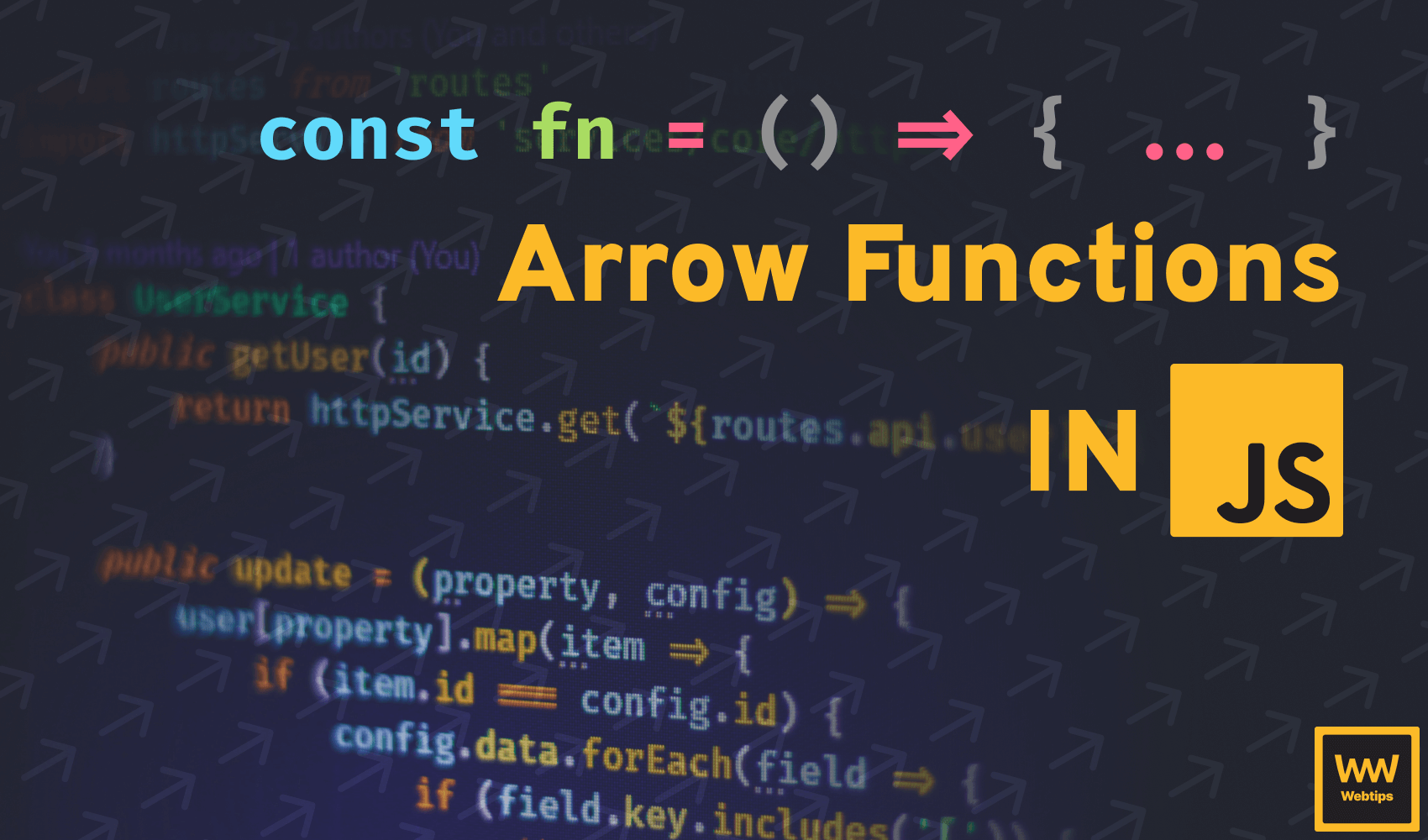
What are Arrow Functions in JavaScript?
Arrow functions offer a concise and convenient way of writing functions. Their shorter syntax compared to traditional function expressions is the reason they gained popularity among developers. They can capture lexical values and are simple to use.
In this article, we'll delve deep into arrow functions, covering their syntax, usage, common code patterns, and their limitations, along with their solutions.
The Syntax of Arrow Functions
The syntax of an arrow function consists of a parameter list, followed by the arrow (=>
) and a function body. The function body can either be a single expression or a block of statements enclosed in curly braces.
Here's the basic syntax of an arrow function with a single parameter and a single-line function body:
const name = parameter => returnValue;
When arrow functions are written on a single line, we can omit the return
keyword. The following syntax can be used for an arrow function with multiple parameters and a block statement as the function body:
const name = (parameter1, parameter2) => {
// statements
return value;
};
Benefits of Using Arrow Functions
Arrow functions provide a concise syntax, especially for writing simple functions. They eliminate the need for the function
keyword, parentheses around a single parameter, and curly braces for one-line functions. Here is a code snippet comparing function expression, function declaration, and arrow function:
// Function Expression:
const greet = function() {
return `Hello, ${name}!`;
}
// Function Declaration:
function greetFunction(name) {
return `Hello, ${name}!`;
}
// Arrow Function:
const greetArrow = name => `Hello, ${name}!`;
// Calling the functions
console.log(greetFunction("Alice")); // Output: Hello, Alice!
console.log(greetArrow("Adeola")); // Output: Hello, Adeola!
In the code above, greet
is a function expression, greetFunction
is a function declared using the function
keyword, while greetFunction
is an arrow function declared using the =>
syntax. Both functions achieve the same result of greeting a person with a given name, but they have slightly different syntax.
Lexical this binding
Unlike traditional functions, arrow functions do not have their own this
value. Instead, they inherit the this
value from the surrounding context. This behavior can be useful when dealing with callbacks or nested functions. Take the following as an example:
const shape = {
radius: 10,
perimeter: () => 2 * Math.PI * this.radius
};
// This will return NaN
console.log(shape.perimeter());
The perimeter
method will produce NaN
, as this
is pointing to the enclosing scope of the shape
object. If we run the above example in a browser and log out the value of this
, we'll see it's pointing to the window
object. Although the following example is not a recommended approach, it'll fix the perimeter
function:
window.radius = 10;
const shape = {
perimeter: () => 2 * Math.PI * this.radius
};
// This will return ~63
console.log(shape.perimeter());

Practical Example
Let's see some practical examples of arrow functions in action. Here's how you can build a simple calculator using arrow functions:
// Arrow function for addition
const add = (num1, num2) => num1 + num2;
// Arrow function for subtraction
const subtract = (num1, num2) => num1 - num2;
// Arrow function for multiplication
const multiply = (num1, num2) => num1 * num2;
// Arrow function for division
const divide = (num1, num2) => {
if (num2 === 0) {
return "Cannot divide by zero";
}
return num1 / num2;
};
// Example usage
console.log(add(8, 3)); // Output: 11
console.log(subtract(3, 3)); // Output: 0
console.log(multiply(5, 7)); // Output: 35
console.log(divide(24, 8)); // Output: 3
console.log(divide(10, 2)); // Output: 5
Limitations of Arrow Functions
Arrow functions also have some limitations compared to regular functions. When working with them, keep the following limitations in mind:
- Arrow functions cannot be used with the
new
keyword to create objects. They lack the necessary internal functionality to act as constructors for new instances. - Arrow functions do not have their own
arguments
object. In regular function declarations, you can access thearguments
object to access all passed arguments, even if they're not explicitly defined in the function parameter list. Arrow functions, however, inherit the arguments object from their containing scope, which may not always behave as expected. - Since arrow functions don't bind their own
this
, we cannot usecall
,apply
, andbind
functions in conjunction with arrow functions to modify the context of thethis
keyword.
// #1: Cannot be used with constructors
const Calculator = () => { ... };
const calculator = new Calculator(); // Calculator is not a constructor
// #2: They don't have an arguments object
const parameters = (a, b) => console.log(arguments);
// #3: Cannot be used with call, apply or bind
const greet = () => `Hello, ${this.name}!`;
greet.call({ name: 'Alice' }); // Output: Hello, undefined!
greet.apply({ name: 'Alice' }); // Output: Hello, undefined!
Conclusion
In conclusion, arrow functions in JavaScript offer a concise and elegant way to write functions, making your code cleaner and more readable.
Their shorter syntax and lexical this
binding make them a valuable addition to your JavaScript toolkit. When using them, keep the above limitations in mind to avoid running into unexpected errors.
Happy coding!
Access 100+ interactive lessons
Unlimited access to hundreds of tutorials
Prepare for technical interviews