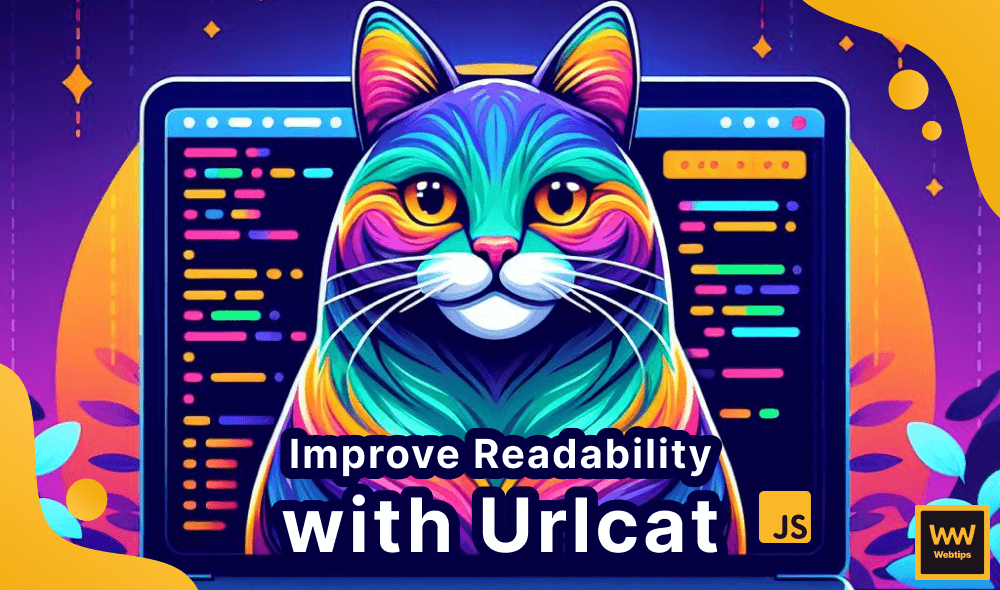
An Introduction to Urlcat
I found this tiny library very different from other libraries because of its unique features; it's just a PnP (plug-and-play). You can use its URL API to build your URLs, and it'll work perfectly without installing any dependencies from @types
.
What is Urlcat?
Urlcat is a very small JavaScript library that enables the building of URLs very conveniently. You don't need to spend hours on documentation or learning complicated patterns; it also helps build URLs easily. It's a library for concatenating URLs.
Features of Urlcat
- Friendly API: This means that urlcat allows software integration by making its application functionality more straightforward and more secure for users.
- One dependency: Urlcat makes use of only one dependency formally known as a query string (qs), a library with some added security.
- It provides TypeScript types: This means that it can work with some of the simplest units of data: numbers, strings, structures, boolean values, and others.
- 0.6 KB minified and gzipped: The size is determined by the dependencies' (direct or transitive) contribution to urlcat's size.
Why We Should Use Urlcat
When there is a need to add vital parameters to the URL, we usually need to make use of a template literal like below:
const API_URL = 'https://api.example.com/'
function getUserPosts(id, blogId, limit, offset) {
const requestUrl = `${API_URL}/users/${id}/blogs/${blogId}/posts?limit=${limit}&offset=${offset}`
// send HTTP request
}
Looking at the code above closely, you'll notice that there are many embedded values and duplicate slashes, which makes this code very difficult to read and understand. This can be corrected using Urlcat to make the code more accessible and simple to read and understand:
const API_URL = 'https://api.example.com/';
function getUserPosts(id, limit, offset) {
const requestUrl = urlcat(API_URL, '/users/:id/posts', { id, limit, offset })
// send HTTP request
}
Now that it has been simplified using this tiny JavaScript library, users can read, write, and clearly understand the code.

How to Install Urlcat
This library can be installed via npm. You can install it by running npm i --save urlcat
inside your terminal. When installed, it'll show up in your package.json
file:
{
"name": "js",
"version": "0.0.0",
"private": true,
"dependencies": {
"urlcat": "2.0.4"
}
}
How to Use Urlcat
Urlcat has two uses: it can be used with Node and Deno. Let's explore its usage with Node first. When building full URLs, these are the most commonly used cases:
import urlcat from 'urlcat'
// To use any utility function, use the import below:
import { query, subst, join } from 'urlcat'
// If you're using CJS, use one of the options below:
const urlcat = require('urlcat').default
const { query, subst, join } = require('urlcat')
Some versions of TypeScript are not supported, but TypeScript 2.1 and above are formally endorsed. When using TypeScript, you don't need to install anything.
A utility function in urlcat
is a function that performs a specific task to support the main program. It performs tasks such as validating input and formatting output.
Utility functions in urlcat
are designed to make the application more efficient and easier to maintain by breaking down complex tasks into smaller, reusable components. To perform a specific task in urlcat
, use one of its utility functions below:
// Use `query` for building query strings:
query({ query: 'string', with: 'params' }) -> 'query=string&with=params'
// Use `subst` for replacing values in template strings:
subst(`/users/:id`, { id: 1 }) -> '/users/1'
// Use `join` for concatenating two strings together with one separator:
join('one', ',', 'two') -> 'one,two'
join('one,', ',', ',two') -> 'one,two'
Usage with Deno
Deno is a next-generation JavaScript runtime that also supports TypeScript natively. You can use urlcat
in Deno using the following code:
import urlcat from 'https://deno.land/x/urlcat/src/index.ts'
console.log(urlcat('https://api.foo.com', ':name', { id: 25, name: 'knpwrs' }))
Conclusion
Building projects should be quick and easier to maintain with urlcat
. Ensure you're using the supported versions of Node and Typescript to avoid problems while working. It's plug-and-play, meaning You don't need to learn complicated patterns or spend hours on documentation; just install Urlcat to make complex code blocks easier to understand.
Access 100+ interactive lessons
Unlimited access to hundreds of tutorials
Prepare for technical interviews