How to Disable Buttons in React Based on Input Fields
To disable buttons in React based on the value of an input field, use the disabled
prop with a value pointing to a useState
hook. In the example below, we only enable the button once the email input field has some value:
Start typing in the input to enable the button.
- Line 4: We can achieve this functionality by introducing a new
useState
hook. - Line 11: We set the value of the input field to our state using an
onChange
event. - Line 14: This negated value is then used for the
disabled
prop, telling React to only enable the button if the field is not empty. Note that empty strings are evaluated asfalse
in JavaScript.
Disable Buttons Based on Multiple Inputs
We can do the same with multiple input fields too; we just need to combine different useState
variables for the disabled
prop. For example:
import { useState } from 'react'
export default function App() {
const [email, setEmail] = useState('')
const [name, setName] = useState('')
return (
<div>
<input
type="text"
value={name}
onChange={event => setName(event.target.value)}
/>
<input
type="email"
value={email}
onChange={event => setEmail(event.target.value)}
/>
<button disabled={!email || !name}>Subscribe</button>
</div>
)
}
- Lines 4-5: First, we create the state for the input fields. In this example, we use a
name
andemail
state. - Lines 12, 17: The states are then used as values for the inputs. Using the
onChange
event, we update their values. - Line 20: Using the state variables, we can create a logical OR to check if any of the fields are empty. The
disabled
prop will only befalse
if none of the fields are empty.
This logic can be recreated with any number of inputs to ensure the submit button is only enabled when all input fields are filled.
Disable Buttons After Click
We can also disable buttons after clicking them to prevent multiple clicks by attaching an onClick
event listener to the button and set the currentTarget.disabled
property to true
:
const disableOnClick = event => event.currentTarget.disabled = true
return <button onClick={disableOnClick}>Send</button>
This way, we don't need to check the value of the input fields; the button will be disabled regardless of the number of inputs and their values. This can be useful if you want to prevent multiple form submissions with different values in the same session.

Summary
In short, use the disabled
prop to disable a button in React based on the value of an input field. If you need to disable the button based on the value of multiple input fields, connect multiple state variables using a logical OR. Using the above-mentioned onClick
event listener, we can also disable buttons on click to prevent multiple user actions.
If you are new to React and would like to learn more, be sure to check out our React roadmap to get up and ready with the core concepts of React.
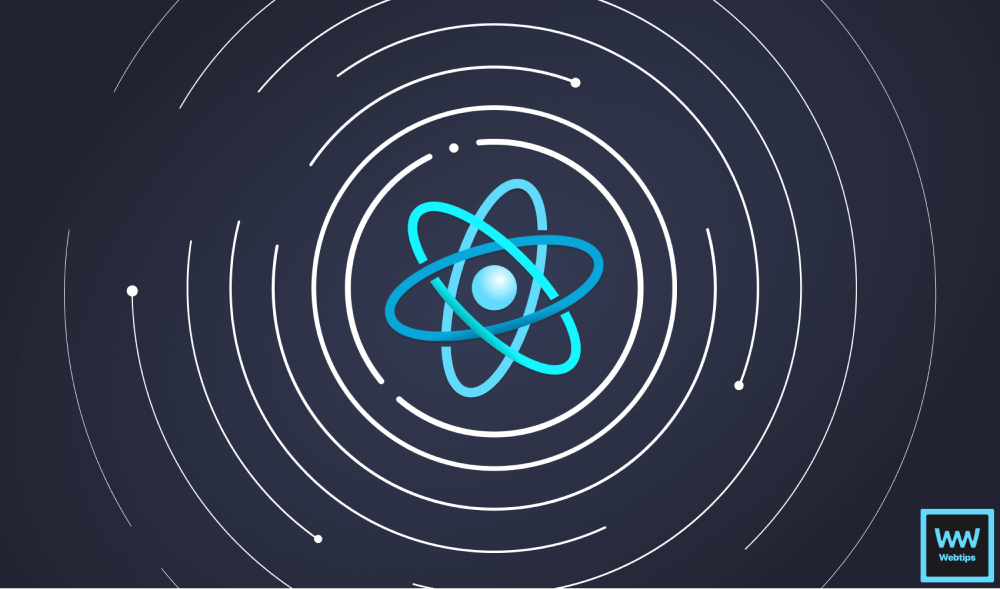
Access 100+ interactive lessons
Unlimited access to hundreds of tutorials
Prepare for technical interviews